WordPress is one of the most popular content management systems in the world, and its flexibility allows developers to add custom functionality as needed. Knowing how to trigger a form submission in WordPress efficiently is a skill that can save time and make your site more user-friendly. In this guide, we’ll explore various methods to trigger form submissions in WordPress and ensure a smoother experience for both you and your website users.
Understanding Form Submission in WordPress
Forms are essential for websites as they enable users to interact with businesses—be it contact forms, signup forms, or survey submissions. By default, WordPress makes it easy to insert and use forms through plugins or custom scripts. However, there are scenarios where you may wish to trigger a form submission programmatically or through custom scripts for added functionality.
Why Might You Need to Trigger a Form Submission?
Before diving into the ‘how’, it’s essential to understand the ‘why’. Some common scenarios include:
- Submitting the form dynamically after validating fields with JavaScript.
- Automatically submitting a form after a pre-determined user action.
- Integrating the form with third-party APIs, requiring customized submission.
- Avoiding unnecessary refreshes to improve the user experience.
Now that you understand the potential use cases, let’s get into how to trigger form submissions effectively.
Methods to Trigger Form Submission
1. Using JavaScript for Client-Side Submission
JavaScript allows you to trigger form submissions directly in the browser without requiring a page refresh. This is especially useful for enhancing UX and speeding up processes.
document.getElementById('form-id').submit();
Here’s how it works:
- Identify your form with a unique ID. For example:
- Add the JavaScript code above to a custom script file or a
<script>
block. - Replace
'form-id'
with your form’s ID, such as'my-form'
. - Trigger the method within an event listener (e.g., a button click).
<form id="my-form"></form>
This approach ensures your form is submitted whenever the method is called.
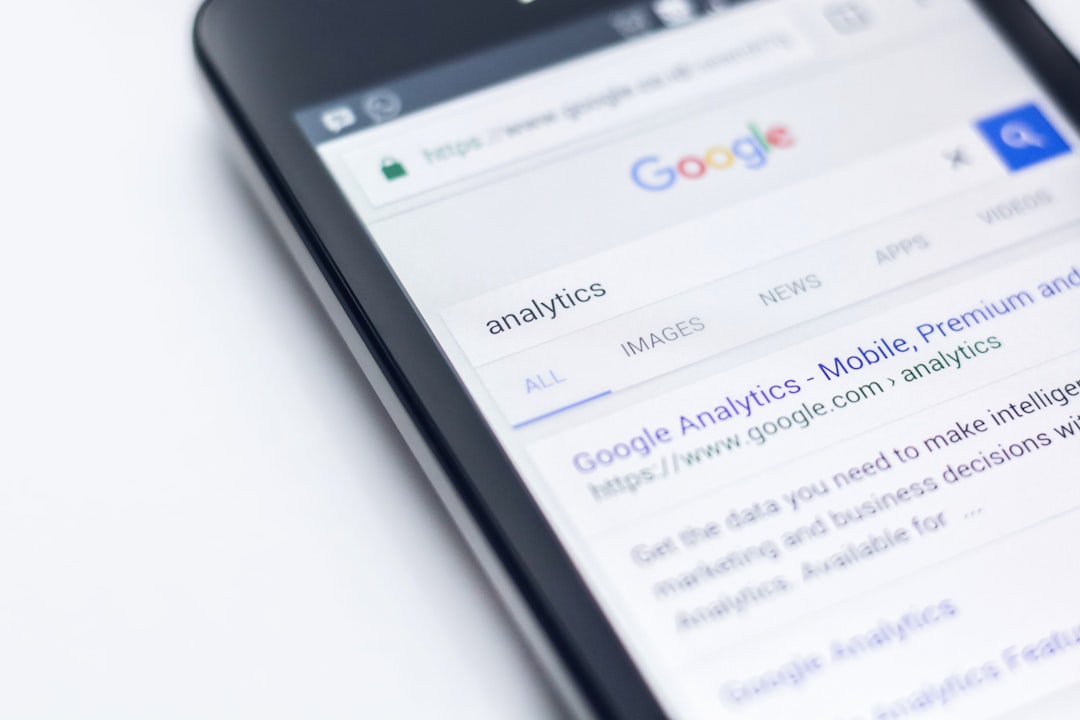
2. Submitting Forms Using WordPress Filters and Hooks
If you’re looking for a server-side solution, WordPress hooks and filters are the way to go. This method is robust, particularly when integrating with plugins like Contact Form 7, Gravity Forms, or WPForms.
Example: Hooking Into a Plugin Submission Action
Consider using a plugin like WPForms. You can use the following hook to customize form handling:
add_action( 'wpforms_process_complete', 'on_form_submission', 10, 4 );
function on_form_submission( $fields, $entry, $form_data, $entry_id ) {
// Your custom logic here
}
- $fields: Submitted form fields.
- $entry: Entry data.
- $form_data: Form metadata.
- $entry_id: Form submission ID.
This PHP logic executes custom code on form submission without disrupting the plugin’s existing functionality.
3. AJAX-Powered Form Submission
A more advanced approach to triggering form submission is by using AJAX to dynamically send data to the server. The benefit of AJAX is that it doesn’t require a full page reload, resulting in a faster and seamless user experience.
Here are the steps:
- Include an AJAX URL in your theme files using
wp_localize_script
. - Use JavaScript (jQuery or vanilla JS) to send the form data to your WordPress backend.
- Create a custom WordPress action hook to process the form data.
Example JavaScript code:
jQuery('#my-form').submit(function(e) {
e.preventDefault(); // Prevent default form submission
var formData = jQuery(this).serialize(); // Serialize form data
jQuery.ajax({
url: ajaxurl, // AJAX URL defined by WordPress
type: 'POST',
data: { action: 'my_form_action', formData: formData },
success: function(response) {
console.log('Form submitted successfully:', response);
},
error: function(error) {
console.error('Form submission error:', error);
}
});
});
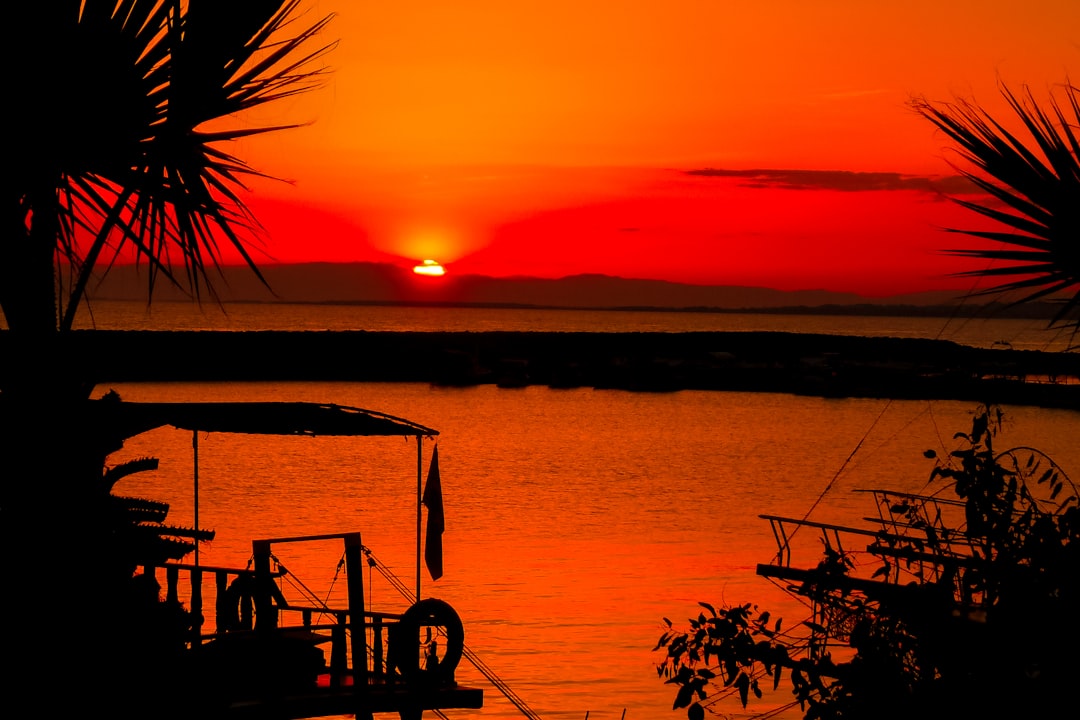
In WordPress, you process the form with a PHP function hooked to the AJAX action:
add_action('wp_ajax_my_form_action', 'handle_form_submission');
add_action('wp_ajax_nopriv_my_form_action', 'handle_form_submission');
function handle_form_submission() {
// Process the form data sent via AJAX
echo "Form data received.";
wp_die();
}
4. Programmatic Submission for Automation
Last but not least, if you ever need to submit a form automatically without user interaction, you can use cURL or similar libraries in PHP. This is typically reserved for highly technical implementations.
Best Practices for Form Submission
When dealing with form submissions, keep the following best practices in mind:
- Validate all inputs on both client and server sides.
- Ensure forms follow accessibility standards for inclusivity.
- Secure submissions using nonces and other authentication measures.
Conclusion
Triggering form submissions in WordPress can open up a range of possibilities for custom functionality. Whether you’re using JavaScript for a client-side solution, WordPress hooks for server-side processing, or combining it all with AJAX, having a clear understanding of the tools and techniques available will help you achieve your goals efficiently.
With the foundation provided in this guide, you’re ready to handle form submissions in WordPress like a pro!